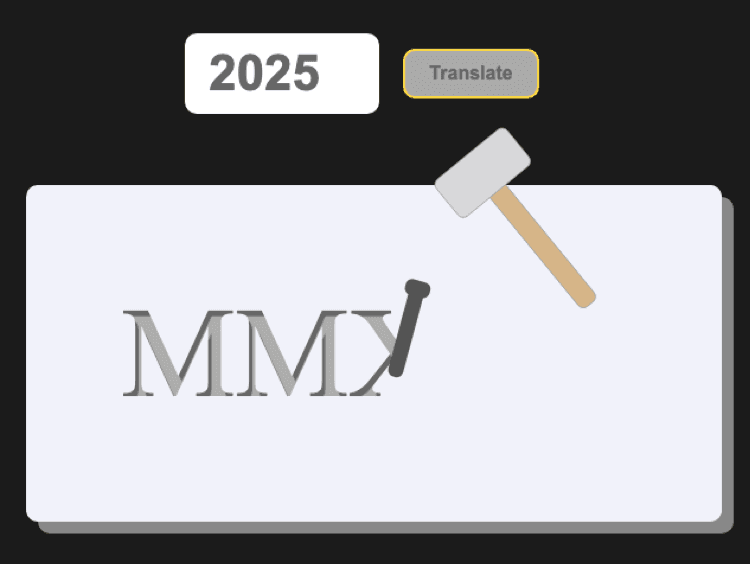
Coding Challenge: Roman Numeral Translator
Published: Apr 2, 2025
In this coding challenge, I made a program that translates modern numbers (Arabic numerals) to Roman Numerals.
Roman numerals are a numeral system that originated in ancient Rome. Numbers are written with combinations of letters from the Latin alphabet, each with a fixed integer value. Roman numerals employ a combination of seven basic symbols: I, V, X, L, C, D, and M, representing the values 1, 5, 10, 50, 100, 500, and 1000, respectively. These symbols are combined and arranged according to specific rules to denote different quantities.
Roman numerals offer a fascinating glimpse into the history of mathematics and how we represent numbers. The modern form of roman numerals with the seven basic symbols described above can only represent numbers from 1 to 3999. They didn’t have a zero. It really makes me wonder how such an impressive civilization was able to be built with a such a number system.
For my program I wrote an algorithm to accept any number between 1 and 3999 and turn it into a Roman numeral text string. It works by subtracting away the biggest roman numeral value you can from your input integer and adding the roman numeral to a string. You work your way through the list of the possible roman numerals from biggest to smallest (including 4s, 9s, 90s, etc. which are considered as a single numeral in the algorithm) until you are left with 0. At that point your string is the roman numeral.
var intToRoman = function(num) {
const symbolVals = { 'M': 1000, 'CM': 900, 'D': 500, 'CD': 400,
'C': 100, 'XC': 90, 'L': 50, 'XL': 40, 'X': 10, 'IX': 9,
'V': 5, 'IV': 4, 'I': 1 }
const symbols = Object.keys(symbolVals)
let roman = ""
let currentSymbolIdx = 0
while (num > 0) {
let currentVal = symbolVals[symbols[currentSymbolIdx]]
if (num - currentVal >= 0) {
roman += symbols[currentSymbolIdx]
num -= currentVal
} else {
currentSymbolIdx += 1
}
}
return roman
};
For my program, I wanted to give the user interface some ancient Roman aesthetics so I gave the project an engraved text effect that is carved into marble or stone. I also made a loading animation that looks like a hammer and chisel carving from left to right into the stone.
You can interact with the project full screen on Codepen: https://codepen.io/fleemaja/full/vEYvNKv. You can also see the project here:
The interface is an HTML form that contains a number input and a “translate” button. The user can increment or decrement the integer value or input a value (an integer between 1 and 3999). I used the javascript event method “preventdefault” to prevent an unnecessary page refresh or call to a server when the html form gets submitted on press of the translate button. The form instead gets “submitted” through a javascript function that updates the value of the roman numeral text that gets “engraved” into the marble block.
I found the engraved effect here: https://www.30secondsofcode.org/css/s/engraved-embossed-text/. This is perfect to create the effect of a Roman marble or stone tablet or monument that has been carved into. This effect is done with just a couple of CSS properties. It sets the text to transparent and then sets the background to clip to the text, so that the background only paints inside the bounds of the text. You then add a text-shadow that is shifted right and down and is blurred slightly. This text shadow also gets clipped by the bounds of the text because apparently the visibility of the text-shadow is still tied to the presence and rendering of the text itself. The text shadow property creates the effect of engraved inset shadows.
Creating the hammer and chisel animation was by far the most challenging part of this project and one of the most frustrating coding experiences I’ve had in a while.
I thought about making this animation with pure CSS, but I got frustrated quickly when trying to do that. I then decided to use simple SVGs (scalable vector graphics) thinking it would be simple to animate them with CSS. I tried messing with CSS animations for hours without success and eventually found a Javascript library called GSAP that is used for complex animations on the web, particularly for things involving timelines of animations. This library was hard to use for me and I found it a little tough to find good examples to learn from. I’ve recently been using AI a lot more to help me code, so if I were re-doing this project now I would get it to teach me how to use this library more easily and thoroughly.
I did a bunch of tinkering and experimentation and eventually got my simple hammer and chisel SVG shapes angled and positioned properly, moving from left to right over a 2 second timeline, making repeated hammering motions (rotating the hammer from an origin point at its handle and moving it up and down a bit), and releasing a dust cloud on every hammer strike. I had trouble getting the hammer and chisel to reset for more animations after completing its initial animation due to some misunderstandings of GSAP, but I was eventually able to get it to reset properly through some tinkering.
To create the illusion that the hammer and chisel were etching into the stone block, I needed to create an animation that revealed the engraved text as if it were being etched from left to right. To do this, I covered the roman numeral engraved text with a rectangle that is the same color as the background. This rectangle is pinned to the right of the marble block and loses width at the same rate that the hammer and chisel are moving from left to right over the marble block, revealing the engraved text underneath it.
My dust cloud animation was also tough to make. I looked around the web for fireworks effects with CSS and other particle effects, but couldn’t find anything that worked well with what I was doing. I ended up adding several little SVG circles to my HTML file to animate with GSAP. They started overlapped under the chisel’s tip and each gets sent outwards at different angles as their opacity dissipates into nothingness, producing a dust cloud impact effect. It’s not exactly realistic, but I think it does a good job conveying the sense of impact of the hammer into the stone.
Overall, this is a simple little program, but the aesthetic touch of the hammer and chisel animation was very tough to make. I have a lot to learn about web animations, whether done with CSS, SVG, or Javascript. I think the hammer and chisel carving into stone is a cool effect for a loading animation and it adds charm to a simple little program. I’m looking forward to trying to hone my skills at creating more charming effects that add some flavor and fun to my coding projects in the future.